Kotzilla Platform
Debug your Kotlin apps and SDKs right in your IDE
Built for Kotlin and Koin
The Kotzilla Platform is a debugging tool for Kotlin, developed by the creators of the Koin framework. The platform allows you to detect and debug both Koin configuration issues and app issues; including performance, complexity, and crashes directly in your IDE.
It uses the Koin container to automatically captures only the essential data needed for debugging, ensuring minimal overhead. You can use it to debug Android apps, KMP apps, and SDK libraries, both during development and in production, with no instrumentation required.
It uses the Koin container to automatically captures only the essential data needed for debugging, ensuring minimal overhead. You can use it to debug Android apps, KMP apps, and SDK libraries, both during development and in production, with no instrumentation required.
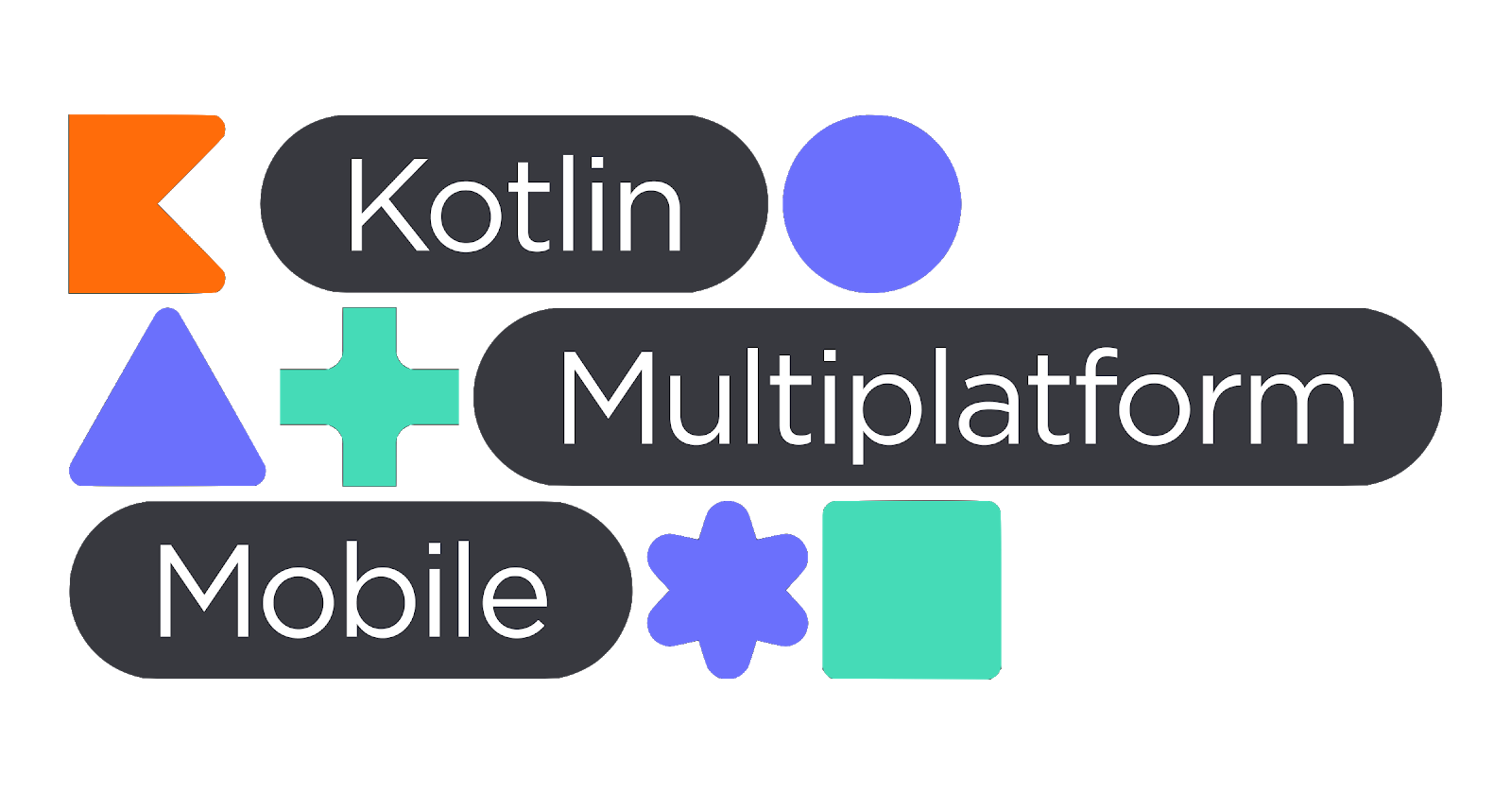